Augmented Reality using ArUco Markers in OpenCV
In this guide, we will explore how to create an AR application using ArUco markers and the OpenCV library in Python.
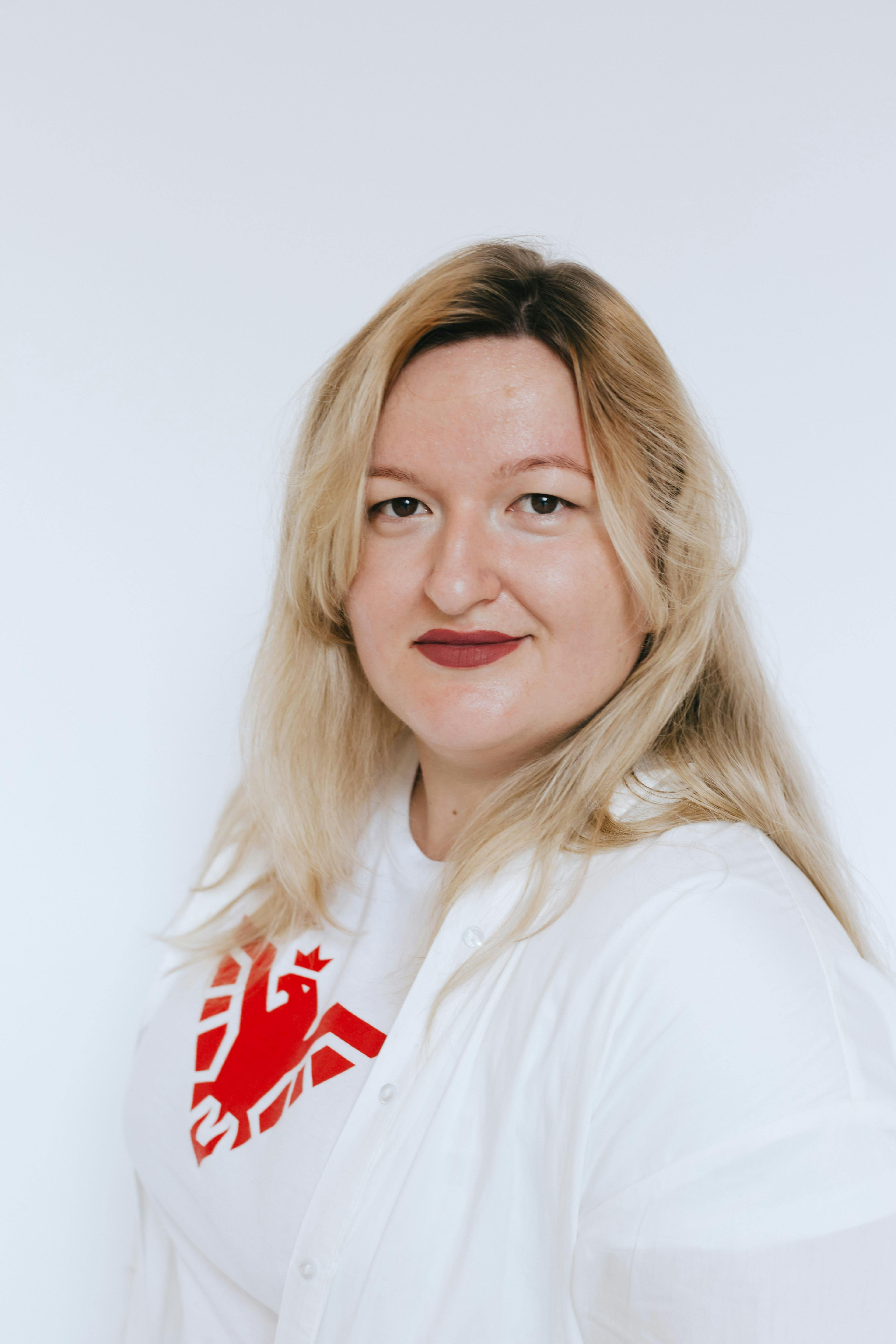
Olena Dontsova
Head of Marketing
25 Oct 2023
7 min read
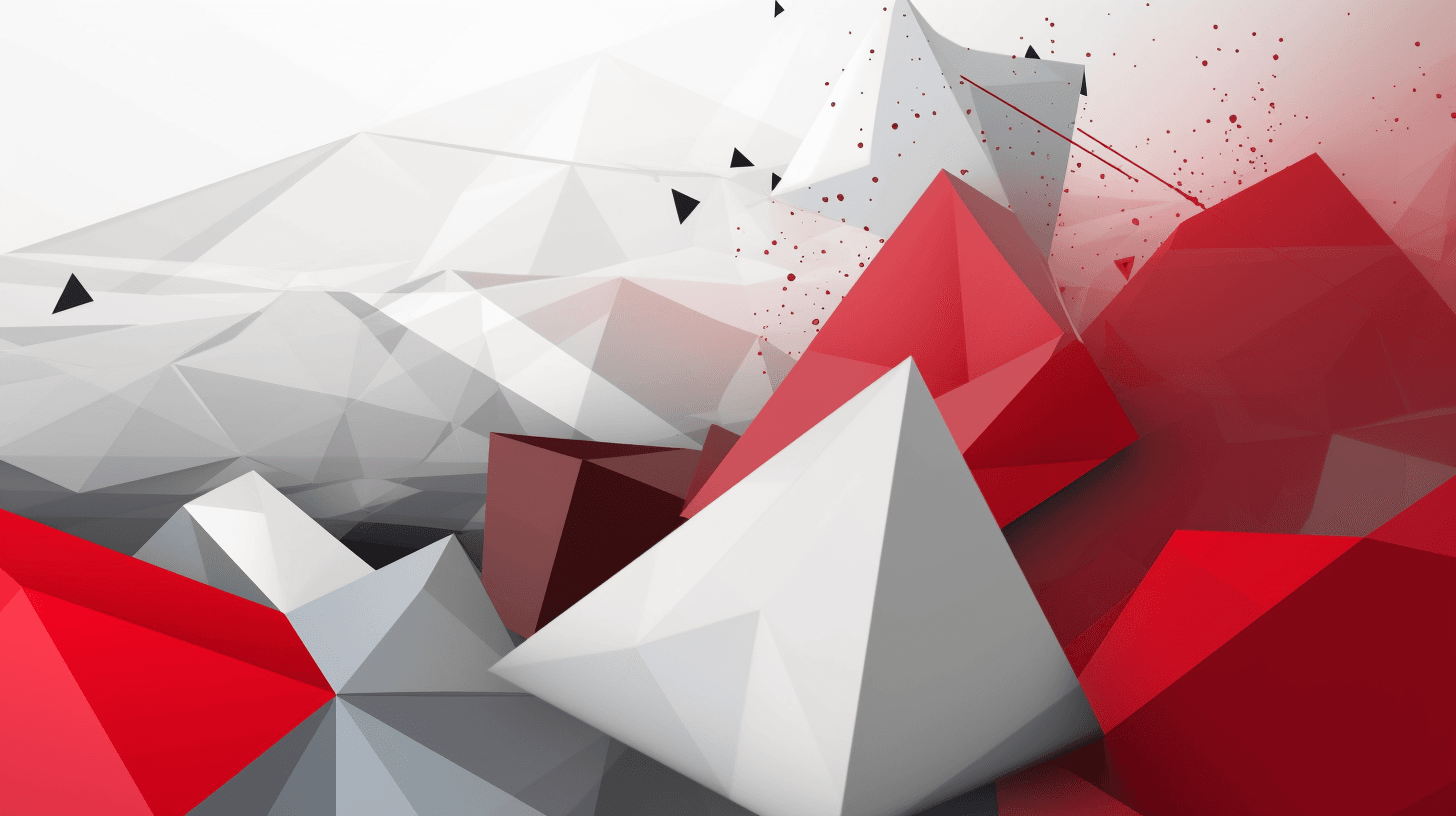
Introduction to Augmented Reality and ArUco Markers
Augmented Reality (AR) has emerged as a transformative technology that bridges the gap between the physical and digital realms. Unlike Virtual Reality (VR), which creates entirely immersive digital environments, AR enhances our real-world experiences by overlaying virtual content onto the physical environment. This fusion of real and digital worlds opens up a multitude of possibilities across various domains, from entertainment and education to healthcare and industrial applications.
In AR, digital information such as images, videos, animations, and 3D models is seamlessly integrated into the user's view of the real world. This integration can take place through devices like smartphones, tablets, smart glasses, and even specialized AR headsets. Imagine being able to see contextual information about landmarks as you travel, visualize furniture in your home before purchasing, or receive step-by-step instructions overlaid on a real object during a repair.

Introducing ArUco Markers and their Significance
One of the key challenges in AR is accurately anchoring digital content to the real world. This is where markers come into play. Markers are specially designed patterns that are easy for computer vision algorithms to detect and recognize. They serve as reference points in the physical environment, allowing AR systems to understand the position and orientation of the user's device relative to these markers.
ArUco markers, short for "Augmented Reality University of Cordoba," are a type of square marker that has gained popularity for marker-based AR applications. These markers possess several qualities that make them ideal for tracking:
Simplicity
ArUco markers are composed of a simple grid of black and white squares, making them easy to generate, print, and detect.
Distinctive Patterns
Each marker has a unique pattern encoded in its arrangement of squares, enabling reliable identification.
Robust Detection
ArUco markers are designed to be detectable even in challenging conditions, such as varying lighting and angles. ArUco markers serve as visual anchors in the environment, allowing AR systems to accurately overlay digital content onto the physical world. When a camera or device captures a scene containing ArUco markers, the AR system can use computer vision techniques to recognize and analyze the markers' positions and orientations. This information forms the basis for aligning and rendering virtual content in a way that seamlessly integrates with the user's view.
Using OpenCV for Augmented Reality
For this tutorial, we will harness the power of OpenCV, a versatile and widely-used open-source computer vision library, to implement Augmented Reality using ArUco markers. OpenCV provides a wealth of functions and tools for image processing, pattern detection, camera calibration, and more. By combining OpenCV's capabilities with ArUco markers, we can create a robust AR experience that detects markers, estimates their poses, and augments reality with virtual content.
In the subsequent sections of this tutorial, we will delve into the details of generating ArUco markers, calibrating the camera, detecting markers, estimating their poses, and finally, overlaying digital content onto the markers. By the end of this guide, you'll have a solid understanding of how to create your own Augmented Reality applications using ArUco markers and OpenCV, opening the door to countless creative possibilities in the world of AR.
Prerequisites
Before you begin, make sure you have the following prerequisites installed:
- Python (3.6 or above)
- OpenCV library (pip install opencv-python)
- Numpy library (pip install numpy)
Generating ArUco Markers
ArUco markers are special patterns that are easy for computer vision algorithms to detect and recognize. Let's start by generating a set of ArUco markers using OpenCV.
python
import cv2
import cv2.aruco as aruco
import numpy as np
# Create a dictionary of ArUco markers
aruco_dict = aruco.Dictionary_get(aruco.DICT_6X6_250)
# Create and save multiple ArUco markers
for i in range(5):
marker_image = aruco.drawMarker(aruco_dict, i, 200)
cv2.imwrite(f"marker_{i}.png", marker_image)
This code generates five ArUco markers and saves them as image files.
Camera Calibration
For accurate marker detection and pose estimation, camera calibration is crucial. Capture several images of a chessboard pattern from different angles and use them to calibrate the camera.
python
# Capture images for camera calibration
# ...
# Perform camera calibration
# ...
# Save calibration parameters
# ...
Marker Detection and Pose Estimation
Let's capture video from the camera and detect ArUco markers in real-time. We'll estimate the position and orientation (pose) of detected markers.
# Initialize camera and marker detector
cap = cv2.VideoCapture(0)
parameters = aruco.DetectorParameters_create()
while True:
ret, frame = cap.read()
# Detect ArUco markers
corners, ids, _ = aruco.detectMarkers(frame, aruco_dict, parameters=parameters)
if ids is not None:
# Draw markers and estimate poses
rvecs, tvecs, _ = aruco.estimatePoseSingleMarkers(corners, 0.05, camera_matrix, dist_coeffs)
for i in range(ids.size):
aruco.drawAxis(frame, camera_matrix, dist_coeffs, rvecs[i], tvecs[i], 0.1)
cv2.imshow("AR using ArUco", frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
This code captures video from the camera, detects ArUco markers, estimates their poses, and visualizes the AR experience by drawing coordinate axes on top of the markers.
Augmenting Reality
Now, let's augment the reality by overlaying virtual content on detected ArUco markers.
# Load virtual content (image or 3D model)
content = cv2.imread("virtual_content.png") # Load your content here
while True:
ret, frame = cap.read()
# Detect ArUco markers
corners, ids, _ = aruco.detectMarkers(frame, aruco_dict, parameters=parameters)
if ids is not None:
for i in range(ids.size):
# Estimate marker pose
rvec, tvec = aruco.estimatePoseSingleMarkers(corners[i], 0.05, camera_matrix, dist_coeffs)
# Project virtual content onto the marker
# ...
# Draw markers
aruco.drawDetectedMarkers(frame, corners, ids)
cv2.imshow("AR using ArUco", frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
In this section, you would load your own virtual content (image or 3D model) and project it onto the markers based on their estimated poses.
Congratulations!
You've learned how to create an Augmented Reality application using ArUco markers and OpenCV. This guide covered generating markers, camera calibration, marker detection, pose estimation, and augmenting reality with virtual content. Feel free to explore further, add interactivity, or experiment with more advanced features.
Remember that Augmented Reality offers endless possibilities for creative applications, and this guide serves as a solid foundation for building your own AR experiences.
References
- OpenCV Documentation: https://docs.opencv.org/4.x/index.html
- ArUco Documentation: https://docs.opencv.org/4.x/d5/dae/tutorial_aruco_detection.html
Feel free to expand on each section and customize the code according to your needs. This comprehensive guide should provide readers with a deep understanding of how to implement Augmented Reality using ArUco markers and OpenCV.